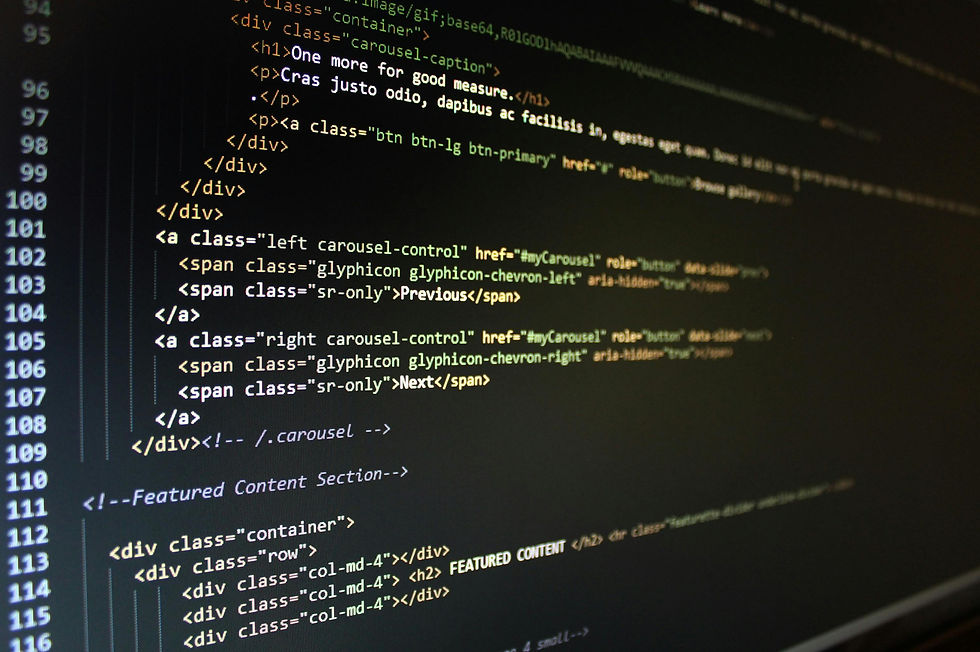
Eoin Butler
5 Jul 2024
A basic beginner guide to understanding XPath and how to use it.
Understanding XPath: A Basic Guide
XPath, short for XML Path Language, is a query language for selecting nodes from an XML document. It can also be used with HTML and is widely utilized in web scraping, testing frameworks, and any application requiring precise navigation of XML or HTML data. This guide aims to demo XPath concepts and showcase its practical applications through various examples.
What is XPath?
XPath is a syntax for defining parts of an XML document. It provides a way to navigate through elements and attributes in XML or HTML. XPath expressions are used to locate and process content in XML documents, functioning similarly to how SQL is used for databases.
Basic Syntax
XPath uses path expressions to navigate through the nodes in an XML document. These expressions can be very simple or extremely complex, depending on the requirements. The primary components of XPath expressions are:
Nodes: The basic units of XPath queries, including elements, attributes, text, etc.
Axes: Define the tree relationship between nodes.
Predicates: Conditions to filter nodes.
Common XPath Expressions
Selecting Nodes
'/' Selects from the root node.
'//' Selects nodes in the document from the current node that match the selection, regardless of where they are.
'.' Selects the current node.
'..' Selects the parent of the current node.
'@' Selects attributes.
Basic Examples
<bookstore>
<book>
<title lang="en">Harry Potter</title>
<author>J K. Rowling</author>
<year>2005</year>
</book>
<book>
<title lang="fr">Le Petit Prince</title>
<author>Antoine de Saint-Exupéry</author>
<year>1943</year>
</book>
</bookstore>
'/bookstore' Selects the root element <bookstore>.
'/bookstore/book' Selects all <book> elements under <bookstore>.
'//title' Selects all <title> elements in the document.
'/bookstore/book[1]' Selects the first <book> element.
'/bookstore/book[last()]' Selects the last <book> element.
'/bookstore/book[position() < 3]' Selects the first two <book> elements.
'//title[@lang='en']' Selects all <title> elements with a lang attribute value of en.
Advanced XPath Concepts
Axes
Axes are used to navigate the hierarchical structure of an XML document. Some common axes include:
child: Selects children of the current node.
parent: Selects the parent of the current node.
ancestor: Selects all ancestors (parent, grandparent, etc.) of the current node.
descendant: Selects all descendants (children, grandchildren, etc.) of the current node.
following-sibling: Selects all siblings after the current node.
/bookstore/book/title/ancestor::bookstore
This selects the <bookstore> element that is an ancestor of the <title> element.
Predicates
Predicates are used to find specific nodes or a node that contains a specific value.
/bookstore/book[author='J K. Rowling']
This selects the <book> element where the <author> is J K. Rowling.
Practical Examples
Web Scraping with XPath
Suppose you have the following HTML structure and you want to extract data from it:
<html>
<body>
<div id="main">
<h1>Bookstore</h1>
<div class="book">
<span class="title">Harry Potter</span>
<span class="author">J K. Rowling</span>
</div>
<div class="book">
<span class="title">Le Petit Prince</span>
<span class="author">Antoine de Saint-Exupéry</span>
</div>
</div>
</body>
</html>
To extract the titles and authors using XPath:
//div[@class='book']/span[@class='title']
//div[@class='book']/span[@class='author']
Testing Frameworks
XPath is frequently used in testing frameworks like Selenium to locate elements on a webpage for automation tasks. For example, in Selenium WebDriver (Python):
from selenium import webdriver
driver = webdriver.Chrome()
driver.get('http://example.com')
title = driver.find_element_by_xpath("//div[@class='book']/span[@class='title']")
author = driver.find_element_by_xpath("//div[@class='book']/span[@class='author']")
print(f"Title: {title.text}, Author: {author.text}")
Conclusion
XPath is a powerful and versatile language for navigating XML and HTML documents. By understanding its basic syntax, axes, and predicates, you can effectively query and manipulate XML/HTML data. Whether you're scraping web content or automating tests, mastering XPath will enhance your ability to work with structured documents.